KIO::WorkerBase
#include <KIO/WorkerBase>
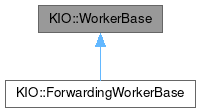
Public Types | |
enum | ButtonCode { Ok = 1 , Cancel = 2 , PrimaryAction = 3 , SecondaryAction = 4 , Continue = 5 } |
enum | MessageBoxType { QuestionTwoActions = 1 , WarningTwoActions = 2 , WarningContinueCancel = 3 , WarningTwoActionsCancel = 4 , Information = 5 , WarningContinueCancelDetailed = 10 } |
Public Member Functions | |
WorkerBase (const QByteArray &protocol, const QByteArray &poolSocket, const QByteArray &appSocket) | |
void | addTemporaryAuthorization (const QString &action) |
MetaData | allMetaData () const |
virtual void | appConnectionMade () |
bool | cacheAuthentication (const AuthInfo &info) |
void | canResume () |
bool | canResume (KIO::filesize_t offset) |
bool | checkCachedAuthentication (AuthInfo &info) |
virtual WorkerResult | chmod (const QUrl &url, int permissions) |
virtual WorkerResult | chown (const QUrl &url, const QString &owner, const QString &group) |
virtual WorkerResult | close () |
virtual void | closeConnection () |
KConfigGroup * | config () |
bool | configValue (const QString &key, bool defaultValue) const |
QString | configValue (const QString &key, const QString &defaultValue=QString()) const |
int | configValue (const QString &key, int defaultValue) const |
int | connectTimeout () |
void | connectWorker (const QString &path) |
virtual WorkerResult | copy (const QUrl &src, const QUrl &dest, int permissions, JobFlags flags) |
void | data (const QByteArray &data) |
void | dataReq () |
virtual WorkerResult | del (const QUrl &url, bool isfile) |
void | disconnectWorker () |
void | dispatchLoop () |
void | errorPage () |
void | exit () |
virtual WorkerResult | fileSystemFreeSpace (const QUrl &url) |
virtual WorkerResult | get (const QUrl &url) |
bool | hasMetaData (const QString &key) const |
void | infoMessage (const QString &msg) |
virtual WorkerResult | listDir (const QUrl &url) |
void | listEntries (const UDSEntryList &_entry) |
void | listEntry (const UDSEntry &entry) |
void | lookupHost (const QString &host) |
QMap< QString, QVariant > | mapConfig () const |
int | messageBox (const QString &text, MessageBoxType type, const QString &title=QString(), const QString &primaryActionText=QString(), const QString &secondaryActionText=QString(), const QString &dontAskAgainName=QString()) |
int | messageBox (MessageBoxType type, const QString &text, const QString &title=QString(), const QString &primaryActionText=QString(), const QString &secondaryActionText=QString()) |
QString | metaData (const QString &key) const |
void | mimeType (const QString &_type) |
virtual WorkerResult | mimetype (const QUrl &url) |
virtual WorkerResult | mkdir (const QUrl &url, int permissions) |
virtual WorkerResult | open (const QUrl &url, QIODevice::OpenMode mode) |
virtual WorkerResult | openConnection () |
int | openPasswordDialog (KIO::AuthInfo &info, const QString &errorMsg=QString()) |
void | position (KIO::filesize_t _pos) |
void | processedSize (KIO::filesize_t _bytes) |
int | proxyConnectTimeout () |
virtual WorkerResult | put (const QUrl &url, int permissions, JobFlags flags) |
virtual WorkerResult | read (KIO::filesize_t size) |
int | readData (QByteArray &buffer) |
int | readTimeout () |
void | redirection (const QUrl &_url) |
KRemoteEncoding * | remoteEncoding () |
virtual WorkerResult | rename (const QUrl &src, const QUrl &dest, JobFlags flags) |
virtual void | reparseConfiguration () |
PrivilegeOperationStatus | requestPrivilegeOperation (const QString &operationDetails) |
int | responseTimeout () |
virtual WorkerResult | seek (KIO::filesize_t offset) |
void | sendAndKeepMetaData () |
void | sendMetaData () |
virtual void | setHost (const QString &host, quint16 port, const QString &user, const QString &pass) |
void | setIncomingMetaData (const KIO::MetaData &metaData) |
void | setMetaData (const QString &key, const QString &value) |
virtual WorkerResult | setModificationTime (const QUrl &url, const QDateTime &mtime) |
void | setTimeoutSpecialCommand (int timeout, const QByteArray &data=QByteArray()) |
virtual WorkerResult | special (const QByteArray &data) |
void | speed (unsigned long _bytes_per_second) |
int | sslError (const QVariantMap &sslData) |
virtual WorkerResult | stat (const QUrl &url) |
void | statEntry (const UDSEntry &_entry) |
virtual WorkerResult | symlink (const QString &target, const QUrl &dest, JobFlags flags) |
void | totalSize (KIO::filesize_t _bytes) |
virtual WorkerResult | truncate (KIO::filesize_t size) |
void | truncated (KIO::filesize_t _length) |
int | waitForAnswer (int expected1, int expected2, QByteArray &data, int *pCmd=nullptr) |
int | waitForHostInfo (QHostInfo &info) |
void | warning (const QString &msg) |
bool | wasKilled () const |
virtual void | worker_status () |
void | workerStatus (const QString &host, bool connected) |
virtual WorkerResult | write (const QByteArray &data) |
void | written (KIO::filesize_t _bytes) |
Detailed Description
WorkerBase is the class to use to implement a worker - simply inherit WorkerBase in your worker.
A call to foo() results in a call to slotFoo() on the other end.
Note that a kioworker doesn't have a Qt event loop. When idle, it's waiting for a command on the socket that connects it to the application. So don't expect a kioworker to react to D-Bus signals for instance. KIOWorkers are short-lived anyway, so any kind of watching or listening for notifications should be done elsewhere, for instance in a kded module (see kio_desktop's desktopnotifier.cpp for an example).
If a kioworker needs a Qt event loop within the implementation of one method, e.g. to wait for an asynchronous operation to finish, that is possible, using QEventLoop.
- Since
- 5.96
Definition at line 81 of file workerbase.h.
Member Enumeration Documentation
◆ ButtonCode
Button codes.
Should be kept in sync with KMessageBox::ButtonCode
Enumerator | |
---|---|
PrimaryAction |
|
SecondaryAction |
|
Definition at line 237 of file workerbase.h.
◆ MessageBoxType
Type of message box.
Should be kept in sync with KMessageBox::DialogType.
Enumerator | |
---|---|
QuestionTwoActions |
|
WarningTwoActions |
|
WarningTwoActionsCancel |
|
Definition at line 223 of file workerbase.h.
Constructor & Destructor Documentation
◆ WorkerBase()
KIO::WorkerBase::WorkerBase | ( | const QByteArray & | protocol, |
const QByteArray & | poolSocket, | ||
const QByteArray & | appSocket ) |
Definition at line 18 of file workerbase.cpp.
Member Function Documentation
◆ addTemporaryAuthorization()
void KIO::WorkerBase::addTemporaryAuthorization | ( | const QString & | action | ) |
Adds action
to the list of PolicyKit actions which the worker is authorized to perform.
- Parameters
-
action the PolicyKit action
Definition at line 431 of file workerbase.cpp.
◆ allMetaData()
MetaData KIO::WorkerBase::allMetaData | ( | ) | const |
for ForwardingWorkerBase Contains all metadata (but no config) sent by the application to the worker.
Definition at line 50 of file workerbase.cpp.
◆ appConnectionMade()
|
virtual |
Application connected to the worker.
Called when an application has connected to the worker. Mostly only useful when you want to e.g. send metadata to the application once it connects.
Definition at line 196 of file workerbase.cpp.
◆ cacheAuthentication()
bool KIO::WorkerBase::cacheAuthentication | ( | const AuthInfo & | info | ) |
Caches info
in a persistent storage like KWallet.
Note that calling openPasswordDialogV2 does not store passwords automatically for you (and has not since kdelibs 4.7).
Here is a simple example of how to use cacheAuthentication:
- Parameters
-
info See AuthInfo.
- Returns
true
ifinfo
was successfully cached.
Definition at line 378 of file workerbase.cpp.
◆ canResume() [1/2]
void KIO::WorkerBase::canResume | ( | ) |
Call this at the beginning of get(), if the "range-start" metadata was set and returning byte ranges is implemented by this protocol.
Definition at line 115 of file workerbase.cpp.
◆ canResume() [2/2]
bool KIO::WorkerBase::canResume | ( | KIO::filesize_t | offset | ) |
Call this at the beginning of put(), to give the size of the existing partial file, if there is one.
The offset
argument notifies the other job (the one that gets the data) about the offset to use. In this case, the boolean returns whether we can indeed resume or not (we can't if the protocol doing the get() doesn't support setting an offset)
Definition at line 353 of file workerbase.cpp.
◆ checkCachedAuthentication()
bool KIO::WorkerBase::checkCachedAuthentication | ( | AuthInfo & | info | ) |
Checks for cached authentication based on parameters given by info
.
Use this function to check if any cached password exists for the URL given by info
. If AuthInfo::realmValue
and/or AuthInfo::verifyPath
flag is specified, then they will also be factored in determining the presence of a cached password. Note that Auth::url
is a required parameter when attempting to check for cached authorization info. Here is a simple example:
- Parameters
-
info See AuthInfo.
- Returns
true
if cached Authorization is found, false otherwise.
Definition at line 373 of file workerbase.cpp.
◆ chmod()
|
virtual |
Change permissions on url
.
The worker emits ERR_DOES_NOT_EXIST or ERR_CANNOT_CHMOD
Reimplemented in KIO::ForwardingWorkerBase.
Definition at line 298 of file workerbase.cpp.
◆ chown()
|
virtual |
Change ownership of url
.
The worker emits ERR_DOES_NOT_EXIST or ERR_CANNOT_CHOWN
Definition at line 308 of file workerbase.cpp.
◆ close()
|
virtual |
◆ closeConnection()
|
virtual |
Closes the connection (forced).
Called when the application disconnects the worker to close any open network connections.
When the worker was operating in connection-oriented mode, it should reset itself to connectionless (default) mode.
Definition at line 209 of file workerbase.cpp.
◆ config()
KConfigGroup * KIO::WorkerBase::config | ( | ) |
Returns a configuration object to query config/meta-data information from.
The application provides the worker with all configuration information relevant for the current protocol and host.
- Note
- Since 5.64 prefer to use mapConfig() or one of the configValue(...) overloads.
- Todo
- Find replacements for the other current usages of this method.
Definition at line 80 of file workerbase.cpp.
◆ configValue() [1/3]
bool KIO::WorkerBase::configValue | ( | const QString & | key, |
bool | defaultValue ) const |
Returns a bool from the config/meta-data information.
Definition at line 65 of file workerbase.cpp.
◆ configValue() [2/3]
QString KIO::WorkerBase::configValue | ( | const QString & | key, |
const QString & | defaultValue = QString() ) const |
Returns a QString from the config/meta-data information.
Definition at line 75 of file workerbase.cpp.
◆ configValue() [3/3]
int KIO::WorkerBase::configValue | ( | const QString & | key, |
int | defaultValue ) const |
Returns an int from the config/meta-data information.
Definition at line 70 of file workerbase.cpp.
◆ connectTimeout()
int KIO::WorkerBase::connectTimeout | ( | ) |
- Returns
- timeout value for connecting to remote host.
- Deprecated
- since 6.11, not used.
Definition at line 384 of file workerbase.cpp.
◆ connectWorker()
void KIO::WorkerBase::connectWorker | ( | const QString & | path | ) |
internal function to connect a worker to/ disconnect from either the worker pool or the application
Definition at line 30 of file workerbase.cpp.
◆ copy()
|
virtual |
Copy src
into dest
.
By default, copy() is only called when copying a file from yourproto://host/path to yourproto://host/otherpath.
If you set copyFromFile=true then copy() will also be called when moving a file from file:///path to yourproto://host/otherpath. Otherwise such a copy would have to be done the slow way (get+put). See also KProtocolManager::canCopyFromFile().
If you set copyToFile=true then copy() will also be called when moving a file from yourproto: to file:. See also KProtocolManager::canCopyToFile().
If the worker returns an error ERR_UNSUPPORTED_ACTION, the job will ask for get + put instead.
If the worker returns an error ERR_FILE_ALREADY_EXIST, the job will ask for a different destination filename.
- Parameters
-
src where to copy the file from (decoded) dest where to copy the file to (decoded) permissions may be -1. In this case no special permission mode is set, and the owner and group permissions are not preserved. flags We support Overwrite here
Don't forget to set the modification time of dest
to be the modification time of src
.
Reimplemented in KIO::ForwardingWorkerBase.
Definition at line 283 of file workerbase.cpp.
◆ data()
void KIO::WorkerBase::data | ( | const QByteArray & | data | ) |
Sends data in the worker to the job (i.e. in get).
To signal end of data, simply send an empty QByteArray().
- Parameters
-
data the data read by the worker
Definition at line 100 of file workerbase.cpp.
◆ dataReq()
void KIO::WorkerBase::dataReq | ( | ) |
◆ del()
|
virtual |
Delete a file or directory.
- Parameters
-
url file/directory to delete isfile if true, a file should be deleted. if false, a directory should be deleted.
By default, del() on a directory should FAIL if the directory is not empty. However, if metadata("recurse") == "true", then the worker can do a recursive deletion. This behavior is only invoked if the worker specifies deleteRecursive=true in its protocol file.
Reimplemented in KIO::ForwardingWorkerBase.
Definition at line 288 of file workerbase.cpp.
◆ disconnectWorker()
void KIO::WorkerBase::disconnectWorker | ( | ) |
Definition at line 35 of file workerbase.cpp.
◆ dispatchLoop()
void KIO::WorkerBase::dispatchLoop | ( | ) |
Definition at line 25 of file workerbase.cpp.
◆ errorPage()
void KIO::WorkerBase::errorPage | ( | ) |
Tell that we will only get an error page here.
This means: the data you'll get isn't the data you requested, but an error page (usually HTML) that describes an error.
- Deprecated
- since 6.3, not implemented/used
Definition at line 156 of file workerbase.cpp.
◆ exit()
void KIO::WorkerBase::exit | ( | ) |
Terminate the worker by calling the destructor and then exit()
Definition at line 166 of file workerbase.cpp.
◆ fileSystemFreeSpace()
|
virtual |
Get a filesystem's total and available space.
- Parameters
-
url Url to the filesystem
Definition at line 313 of file workerbase.cpp.
◆ get()
|
virtual |
get, aka read.
- Parameters
-
url the full url for this request. Host, port and user of the URL can be assumed to be the same as in the last setHost() call.
The worker should first "emit" the MIME type by calling mimeType(), and then "emit" the data using the data() method.
The reason why we need get() to emit the MIME type is: when pasting a URL in krunner, or konqueror's location bar, we have to find out what is the MIME type of that URL. Rather than doing it with a call to mimetype(), then the app or part would have to do a second request to the same server, this is done like this: get() is called, and when it emits the MIME type, the job is put on hold and the right app or part is launched. When that app or part calls get(), the worker is magically reused, and the download can now happen. All with a single call to get() in the worker. This mechanism is also described in KIO::get().
Reimplemented in KIO::ForwardingWorkerBase.
Definition at line 233 of file workerbase.cpp.
◆ hasMetaData()
bool KIO::WorkerBase::hasMetaData | ( | const QString & | key | ) | const |
Queries for the existence of a certain config/meta-data entry send by the application to the worker.
Definition at line 55 of file workerbase.cpp.
◆ infoMessage()
void KIO::WorkerBase::infoMessage | ( | const QString & | msg | ) |
Call to signal a message, to be displayed if the application wants to, for instance in a status bar.
Usual examples are "connecting to host xyz", etc.
Definition at line 176 of file workerbase.cpp.
◆ listDir()
|
virtual |
Lists the contents of url
.
The worker should emit ERR_CANNOT_ENTER_DIRECTORY if it doesn't exist, if we don't have enough permissions. You should not list files if the path in url
is empty, but redirect to a non-empty path instead.
Reimplemented in KIO::ForwardingWorkerBase.
Definition at line 228 of file workerbase.cpp.
◆ listEntries()
void KIO::WorkerBase::listEntries | ( | const UDSEntryList & | _entry | ) |
Call this in listDir, each time you have a bunch of entries to report.
- Parameters
-
_entry The UDSEntry containing all of the object attributes.
Definition at line 191 of file workerbase.cpp.
◆ listEntry()
void KIO::WorkerBase::listEntry | ( | const UDSEntry & | entry | ) |
It collects entries and emits them via listEntries when enough of them are there or a certain time frame exceeded (to make sure the app gets some items in time but not too many items one by one as this will cause a drastic performance penalty).
- Parameters
-
entry The UDSEntry containing all of the object attributes.
Definition at line 186 of file workerbase.cpp.
◆ lookupHost()
void KIO::WorkerBase::lookupHost | ( | const QString & | host | ) |
Internally used.
Definition at line 416 of file workerbase.cpp.
◆ mapConfig()
Returns a map to query config/meta-data information from.
The application provides the worker with all configuration information relevant for the current protocol and host.
Use configValue() as shortcut.
Definition at line 60 of file workerbase.cpp.
◆ messageBox() [1/2]
int KIO::WorkerBase::messageBox | ( | const QString & | text, |
MessageBoxType | type, | ||
const QString & | title = QString(), | ||
const QString & | primaryActionText = QString(), | ||
const QString & | secondaryActionText = QString(), | ||
const QString & | dontAskAgainName = QString() ) |
Call this to show a message box from the worker.
- Parameters
-
text Message string. May contain newlines. type type of message box title Message box title. primaryActionText the text for the first button. Ignored for type
Information.secondaryActionText the text for the second button. Ignored for type
WarningContinueCancel, WarningContinueCancelDetailed, Information.dontAskAgainName the name used to store result from 'Do not ask again' checkbox.
- Returns
- a button code, as defined in ButtonCode, or 0 on communication error.
Definition at line 338 of file workerbase.cpp.
◆ messageBox() [2/2]
int KIO::WorkerBase::messageBox | ( | MessageBoxType | type, |
const QString & | text, | ||
const QString & | title = QString(), | ||
const QString & | primaryActionText = QString(), | ||
const QString & | secondaryActionText = QString() ) |
Call this to show a message box from the worker.
- Parameters
-
type type of message box text Message string. May contain newlines. title Message box title. primaryActionText the text for the first button. Ignored for type
Information.secondaryActionText the text for the second button. Ignored for type
WarningContinueCancel, WarningContinueCancelDetailed, Information.
- Returns
- a button code, as defined in ButtonCode, or 0 on communication error.
Definition at line 333 of file workerbase.cpp.
◆ metaData()
Queries for config/meta-data send by the application to the worker.
Definition at line 45 of file workerbase.cpp.
◆ mimeType()
void KIO::WorkerBase::mimeType | ( | const QString & | _type | ) |
Call this in mimetype() and in get(), when you know the MIME type.
See mimetype() about other ways to implement it.
Definition at line 161 of file workerbase.cpp.
◆ mimetype()
|
virtual |
Finds MIME type for one file or directory.
This method should either emit 'mimeType' or it should send a block of data big enough to be able to determine the MIME type.
If the worker doesn't reimplement it, a get will be issued, i.e. the whole file will be downloaded before determining the MIME type on it - this is obviously not a good thing in most cases.
Reimplemented in KIO::ForwardingWorkerBase.
Definition at line 268 of file workerbase.cpp.
◆ mkdir()
|
virtual |
Create a directory.
- Parameters
-
url path to the directory to create permissions the permissions to set after creating the directory (-1 if no permissions to be set) The worker emits ERR_CANNOT_MKDIR if failure.
Reimplemented in KIO::ForwardingWorkerBase.
Definition at line 293 of file workerbase.cpp.
◆ open()
|
virtual |
open.
- Parameters
-
url the full url for this request. Host, port and user of the URL can be assumed to be the same as in the last setHost() call. mode see QIODevice::OpenMode
Definition at line 238 of file workerbase.cpp.
◆ openConnection()
|
virtual |
Opens the connection (forced).
When this function gets called the worker is operating in connection-oriented mode. When a connection gets lost while the worker operates in connection oriented mode, the worker should report ERR_CONNECTION_BROKEN instead of reconnecting. The user is expected to disconnect the worker in the error handler.
Definition at line 204 of file workerbase.cpp.
◆ openPasswordDialog()
int KIO::WorkerBase::openPasswordDialog | ( | KIO::AuthInfo & | info, |
const QString & | errorMsg = QString() ) |
Prompt the user for Authorization info (login & password).
Use this function to request authorization information from the end user. You can also pass an error message which explains why a previous authorization attempt failed. Here is a very simple example:
You can also preset some values like the username, caption or comment as follows:
- Note
- You should consider using checkCachedAuthentication() to see if the password is available in kpasswdserver before calling this function.
-
A call to this function can fail and return
false
, if the password server could not be started for whatever reason. - This function does not store the password information automatically (and has not since kdelibs 4.7). If you want to store the password information in a persistent storage like KWallet, then you MUST call cacheAuthentication.
- See also
- checkCachedAuthentication
- Returns
- a KIO error code: NoError (0), KIO::USER_CANCELED, or other error codes.
Definition at line 328 of file workerbase.cpp.
◆ position()
void KIO::WorkerBase::position | ( | KIO::filesize_t | _pos | ) |
Definition at line 135 of file workerbase.cpp.
◆ processedSize()
void KIO::WorkerBase::processedSize | ( | KIO::filesize_t | _bytes | ) |
Call this during get and copy, once in a while, to give some info about the current state.
Don't emit it in listDir, listEntries speaks for itself.
Definition at line 125 of file workerbase.cpp.
◆ proxyConnectTimeout()
int KIO::WorkerBase::proxyConnectTimeout | ( | ) |
- Returns
- timeout value for connecting to proxy in secs.
- Deprecated
- since 6.11, not used.
Definition at line 391 of file workerbase.cpp.
◆ put()
|
virtual |
put, i.e. write data into a file.
- Parameters
-
url where to write the file permissions may be -1. In this case no special permission mode is set. flags We support Overwrite here. Hopefully, we're going to support Resume in the future, too. If the file indeed already exists, the worker should NOT apply the permissions change to it. The support for resuming using .part files is done by calling canResume().
IMPORTANT: Use the "modified" metadata in order to set the modification time of the file.
- See also
- canResume()
Reimplemented in KIO::ForwardingWorkerBase.
Definition at line 218 of file workerbase.cpp.
◆ read()
|
virtual |
read.
- Parameters
-
size the requested amount of data to read
- See also
- KIO::FileJob::read()
Definition at line 243 of file workerbase.cpp.
◆ readData()
int KIO::WorkerBase::readData | ( | QByteArray & | buffer | ) |
Read data sent by the job, after a dataReq.
- Parameters
-
buffer buffer where data is stored
- Returns
- 0 on end of data, > 0 bytes read < 0 error
Definition at line 363 of file workerbase.cpp.
◆ readTimeout()
int KIO::WorkerBase::readTimeout | ( | ) |
- Returns
- timeout value for read from subsequent data from remote host in secs.
- Deprecated
- since 6.11, not used.
Definition at line 405 of file workerbase.cpp.
◆ redirection()
void KIO::WorkerBase::redirection | ( | const QUrl & | _url | ) |
Call this to signal a redirection.
The job will take care of going to that url.
Definition at line 150 of file workerbase.cpp.
◆ remoteEncoding()
KRemoteEncoding * KIO::WorkerBase::remoteEncoding | ( | ) |
Returns an object that can translate remote filenames into proper Unicode forms.
This encoding can be set by the user.
Definition at line 95 of file workerbase.cpp.
◆ rename()
|
virtual |
Rename oldname
into newname
.
If the worker returns an error ERR_UNSUPPORTED_ACTION, the job will ask for copy + del instead.
Important: the worker must implement the logic "if the destination already exists, error ERR_DIR_ALREADY_EXIST or ERR_FILE_ALREADY_EXIST". For performance reasons no stat is done in the destination before hand, the worker must do it.
By default, rename() is only called when renaming (moving) from yourproto://host/path to yourproto://host/otherpath.
If you set renameFromFile=true then rename() will also be called when moving a file from file:///path to yourproto://host/otherpath. Otherwise such a move would have to be done the slow way (copy+delete). See KProtocolManager::canRenameFromFile() for more details.
If you set renameToFile=true then rename() will also be called when moving a file from yourproto: to file:. See KProtocolManager::canRenameToFile() for more details.
- Parameters
-
src where to move the file from dest where to move the file to flags We support Overwrite here
Reimplemented in KIO::ForwardingWorkerBase.
Definition at line 273 of file workerbase.cpp.
◆ reparseConfiguration()
|
virtual |
Called by the scheduler to tell the worker that the configuration changed (i.e. proxy settings) .
Definition at line 323 of file workerbase.cpp.
◆ requestPrivilegeOperation()
PrivilegeOperationStatus KIO::WorkerBase::requestPrivilegeOperation | ( | const QString & | operationDetails | ) |
Checks with job if privilege operation is allowed.
- Returns
- privilege operation status.
- See also
- PrivilegeOperationStatus
Definition at line 426 of file workerbase.cpp.
◆ responseTimeout()
int KIO::WorkerBase::responseTimeout | ( | ) |
- Returns
- timeout value for read from first data from remote host in seconds.
- Deprecated
- since 6.11, not used.
Definition at line 398 of file workerbase.cpp.
◆ seek()
|
virtual |
seek.
- Parameters
-
offset the requested amount of data to read
- See also
- KIO::FileJob::read()
Definition at line 253 of file workerbase.cpp.
◆ sendAndKeepMetaData()
void KIO::WorkerBase::sendAndKeepMetaData | ( | ) |
Internal function to transmit meta data to the application.
Like sendMetaData() but m_outgoingMetaData will not be cleared. This method is mainly useful in code that runs before the worker is connected to its final job.
Definition at line 90 of file workerbase.cpp.
◆ sendMetaData()
void KIO::WorkerBase::sendMetaData | ( | ) |
Internal function to transmit meta data to the application.
m_outgoingMetaData will be cleared; this means that if the worker is for example put on hold and picked up by a different KIO::Job later the new job will not see the metadata sent before. See kio/DESIGN.krun for an overview of the state progression of a job/worker.
- Warning
- calling this method may seriously interfere with the operation of KIO which relies on the presence of some metadata at some points in time. You should not use it if you are not familiar with KIO and not before the worker is connected to the last job before returning to idle state.
Definition at line 85 of file workerbase.cpp.
◆ setHost()
|
virtual |
Set the host.
Called directly by createWorker and not via the interface.
This method is called whenever a change in host, port or user occurs.
Definition at line 200 of file workerbase.cpp.
◆ setIncomingMetaData()
void KIO::WorkerBase::setIncomingMetaData | ( | const KIO::MetaData & | metaData | ) |
Set the Incoming Meta Data This is only really useful if your worker wants to overwrite the metadata for consumption in other worker functions; this overwrites existing metadata set by the client!
- Parameters
-
metaData metadata to set
- Since
- 5.99
Definition at line 493 of file workerbase.cpp.
◆ setMetaData()
Sets meta-data to be send to the application before the first data() or finished() signal.
Definition at line 40 of file workerbase.cpp.
◆ setModificationTime()
|
virtual |
Sets the modification time for @url.
For instance this is what CopyJob uses to set mtime on dirs at the end of a copy. It could also be used to set the mtime on any file, in theory. The usual implementation on unix is to call utime(path, &myutimbuf). The worker emits ERR_DOES_NOT_EXIST or ERR_CANNOT_SETTIME
Reimplemented in KIO::ForwardingWorkerBase.
Definition at line 303 of file workerbase.cpp.
◆ setTimeoutSpecialCommand()
void KIO::WorkerBase::setTimeoutSpecialCommand | ( | int | timeout, |
const QByteArray & | data = QByteArray() ) |
This function sets a timeout of timeout
seconds and calls special(data) when the timeout occurs as if it was called by the application.
A timeout can only occur when the worker is waiting for a command from the application.
Specifying a negative timeout cancels a pending timeout.
Only one timeout at a time is supported, setting a timeout cancels any pending timeout.
Definition at line 368 of file workerbase.cpp.
◆ special()
|
virtual |
Used for any command that is specific to this worker (protocol).
Examples are : HTTP POST, mount and unmount (kio_file)
- Parameters
-
data packed data; the meaning is completely dependent on the worker, but usually starts with an int for the command number. Document your worker's commands, at least in its header file.
Definition at line 223 of file workerbase.cpp.
◆ speed()
void KIO::WorkerBase::speed | ( | unsigned long | _bytes_per_second | ) |
Call this in get and copy, to give the current transfer speed, but only if it can't be calculated out of the size you passed to processedSize (in most cases you don't want to call it)
Definition at line 145 of file workerbase.cpp.
◆ sslError()
int KIO::WorkerBase::sslError | ( | const QVariantMap & | sslData | ) |
Definition at line 348 of file workerbase.cpp.
◆ stat()
|
virtual |
Finds all details for one file or directory.
The information returned is the same as what listDir returns, but only for one file or directory. Call statEntry() after creating the appropriate UDSEntry for this url.
You can use the "details" metadata to optimize this method to only do as much work as needed by the application. By default details is 2 (all details wanted, including modification time, size, etc.), details==1 is used when deleting: we don't need all the information if it takes too much time, no need to follow symlinks etc. details==0 is used for very simple probing: we'll only get the answer "it's a file or a directory (or a symlink), or it doesn't exist".
Reimplemented in KIO::ForwardingWorkerBase.
Definition at line 213 of file workerbase.cpp.
◆ statEntry()
void KIO::WorkerBase::statEntry | ( | const UDSEntry & | _entry | ) |
Call this from stat() to express details about an object, the UDSEntry customarily contains the atoms describing file name, size, MIME type, etc.
- Parameters
-
_entry The UDSEntry containing all of the object attributes.
Definition at line 181 of file workerbase.cpp.
◆ symlink()
|
virtual |
Creates a symbolic link named dest
, pointing to target
, which may be a relative or an absolute path.
- Parameters
-
target The string that will become the "target" of the link (can be relative) dest The symlink to create. flags We support Overwrite here
Reimplemented in KIO::ForwardingWorkerBase.
Definition at line 278 of file workerbase.cpp.
◆ totalSize()
void KIO::WorkerBase::totalSize | ( | KIO::filesize_t | _bytes | ) |
Call this in get and copy, to give the total size of the file.
Definition at line 120 of file workerbase.cpp.
◆ truncate()
|
virtual |
truncate
- Parameters
-
size size to truncate the file to
- See also
- KIO::FileJob::truncate()
Definition at line 258 of file workerbase.cpp.
◆ truncated()
void KIO::WorkerBase::truncated | ( | KIO::filesize_t | _length | ) |
Definition at line 140 of file workerbase.cpp.
◆ waitForAnswer()
int KIO::WorkerBase::waitForAnswer | ( | int | expected1, |
int | expected2, | ||
QByteArray & | data, | ||
int * | pCmd = nullptr ) |
Wait for an answer to our request, until we get expected1
or expected2
.
- Returns
- the result from readData, as well as the cmd in *pCmd if set, and the data in
data
Definition at line 358 of file workerbase.cpp.
◆ waitForHostInfo()
int KIO::WorkerBase::waitForHostInfo | ( | QHostInfo & | info | ) |
Internally used.
Definition at line 421 of file workerbase.cpp.
◆ warning()
void KIO::WorkerBase::warning | ( | const QString & | msg | ) |
Call to signal a warning, to be displayed in a dialog box.
Definition at line 171 of file workerbase.cpp.
◆ wasKilled()
bool KIO::WorkerBase::wasKilled | ( | ) | const |
If your ioworker was killed by a signal, wasKilled() returns true.
Check it regularly in lengthy functions (e.g. in get();) and return as fast as possible from this function if wasKilled() returns true. This will ensure that your worker destructor will be called correctly.
Definition at line 411 of file workerbase.cpp.
◆ worker_status()
|
virtual |
Called to get the status of the worker.
Worker should respond by calling workerStatus(...)
Definition at line 318 of file workerbase.cpp.
◆ workerStatus()
void KIO::WorkerBase::workerStatus | ( | const QString & | host, |
bool | connected ) |
Used to report the status of the worker.
- Parameters
-
host the worker is currently connected to. (Should be empty if not connected) connected Whether an actual network connection exists.
Definition at line 110 of file workerbase.cpp.
◆ write()
|
virtual |
write.
- Parameters
-
data the data to write
- See also
- KIO::FileJob::write()
Definition at line 248 of file workerbase.cpp.
◆ written()
void KIO::WorkerBase::written | ( | KIO::filesize_t | _bytes | ) |
Definition at line 130 of file workerbase.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:02:24 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.