QCA::CertificateRequest
#include <QtCrypto>
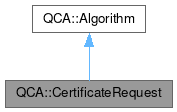
Static Public Member Functions | |
static bool | canUseFormat (CertificateRequestFormat f, const QString &provider=QString()) |
static CertificateRequest | fromDER (const QByteArray &a, ConvertResult *result=nullptr, const QString &provider=QString()) |
static CertificateRequest | fromPEM (const QString &s, ConvertResult *result=nullptr, const QString &provider=QString()) |
static CertificateRequest | fromPEMFile (const QString &fileName, ConvertResult *result=nullptr, const QString &provider=QString()) |
static CertificateRequest | fromString (const QString &s, ConvertResult *result=nullptr, const QString &provider=QString()) |
Additional Inherited Members | |
![]() | |
Algorithm () | |
Algorithm (const QString &type, const QString &provider) | |
Detailed Description
Certificate Request
A CertificateRequest is a unsigned request for a Certificate
Definition at line 1325 of file qca_cert.h.
Constructor & Destructor Documentation
◆ CertificateRequest() [1/4]
QCA::CertificateRequest::CertificateRequest | ( | ) |
Create an empty certificate request.
◆ CertificateRequest() [2/4]
QCA::CertificateRequest::CertificateRequest | ( | const QString & | fileName | ) |
Create a certificate request based on the contents of a file.
- Parameters
-
fileName the file (and path, if necessary) containing a PEM encoded certificate request
◆ CertificateRequest() [3/4]
QCA::CertificateRequest::CertificateRequest | ( | const CertificateOptions & | opts, |
const PrivateKey & | key, | ||
const QString & | provider = QString() ) |
Create a certificate request based on specified options.
- Parameters
-
opts the options to use in the certificate request key the private key that matches the certificate being requested provider the provider to use, if a specific provider is required
◆ CertificateRequest() [4/4]
QCA::CertificateRequest::CertificateRequest | ( | const CertificateRequest & | from | ) |
Standard copy constructor.
- Parameters
-
from the request to copy from
Member Function Documentation
◆ canUseFormat()
|
static |
Test if the certificate request can use a specified format.
- Parameters
-
f the format to test for provider the provider to use, if a specific provider is required
- Returns
- true if the certificate request can use the specified format
◆ challenge()
QString QCA::CertificateRequest::challenge | ( | ) | const |
The challenge associated with this certificate request.
◆ change()
void QCA::CertificateRequest::change | ( | CSRContext * | c | ) |
- Parameters
-
c context (internal)
◆ constraints()
Constraints QCA::CertificateRequest::constraints | ( | ) | const |
The constraints that apply to this certificate request.
- Note
- this only applies to PKCS#10 format certificate requests
◆ format()
CertificateRequestFormat QCA::CertificateRequest::format | ( | ) | const |
the format that this Certificate request is in
◆ fromDER()
|
static |
Import the certificate request from DER.
- Parameters
-
a the array containing the certificate request in DER format result a pointer to a ConvertResult, which if not-null will be set to the conversion status provider the provider to use, if a specific provider is required
- Returns
- the CertificateRequest corresponding to the certificate request in the provided array
- Note
- this only applies to PKCS#10 format certificate requests
◆ fromPEM()
|
static |
Import the certificate request from PEM format.
- Parameters
-
s the string containing the certificate request in PEM format result a pointer to a ConvertResult, which if not-null will be set to the conversion status provider the provider to use, if a specific provider is required
- Returns
- the CertificateRequest corresponding to the certificate request in the provided string
- Note
- this only applies to PKCS#10 format certificate requests
◆ fromPEMFile()
|
static |
Import the certificate request from a file.
- Parameters
-
fileName the name (and path, if required) of the file containing the certificate request in PEM format result a pointer to a ConvertResult, which if not-null will be set to the conversion status provider the provider to use, if a specific provider is required
- Returns
- the CertificateRequest corresponding to the certificate request in the provided string
- Note
- this only applies to PKCS#10 format certificate requests
◆ fromString()
|
static |
Import the CertificateRequest from a string.
- Parameters
-
s the string containing to the certificate request result a pointer to a ConvertResult, which if not-null will be set to the conversion status provider the provider to use, if a specific provider is required
- Returns
- the CertificateRequest corresponding to the certificate request in the provided string
- Note
- this only applies to SPKAC format certificate requests
◆ isCA()
bool QCA::CertificateRequest::isCA | ( | ) | const |
Test if this Certificate Request is for a Certificate Authority certificate.
- Note
- this only applies to PKCS#10 format certificate requests
◆ isNull()
bool QCA::CertificateRequest::isNull | ( | ) | const |
test if the certificate request is empty
- Returns
- true if the certificate request is empty, otherwise false
◆ operator!=()
|
inline |
Inequality operator.
- Parameters
-
other the certificate request to be compared to this certificate request
Definition at line 1473 of file qca_cert.h.
◆ operator=()
CertificateRequest & QCA::CertificateRequest::operator= | ( | const CertificateRequest & | from | ) |
Standard assignment operator.
- Parameters
-
from the request to assign from
◆ operator==()
bool QCA::CertificateRequest::operator== | ( | const CertificateRequest & | csr | ) | const |
Test for equality of two certificate requests.
- Parameters
-
csr the certificate request to be compared to this certificate request
- Returns
- true if the two certificate requests are the same
◆ pathLimit()
int QCA::CertificateRequest::pathLimit | ( | ) | const |
The path limit for the certificate in this Certificate Request.
- Note
- this only applies to PKCS#10 format certificate requests
◆ policies()
QStringList QCA::CertificateRequest::policies | ( | ) | const |
The policies that apply to this certificate request.
- Note
- this only applies to PKCS#10 format certificate requests
◆ signatureAlgorithm()
SignatureAlgorithm QCA::CertificateRequest::signatureAlgorithm | ( | ) | const |
The algorithm used to make the signature on this certificate request.
◆ subjectInfo()
CertificateInfo QCA::CertificateRequest::subjectInfo | ( | ) | const |
Information on the subject of the certificate being requested.
- Note
- this only applies to PKCS#10 format certificate requests
- See also
- subjectInfoOrdered for a version that maintains order in the subject information.
◆ subjectInfoOrdered()
CertificateInfoOrdered QCA::CertificateRequest::subjectInfoOrdered | ( | ) | const |
Information on the subject of the certificate being requested, as an ordered list (QList of CertificateInfoPair).
- Note
- this only applies to PKCS#10 format certificate requests
- See also
- subjectInfo for a version that does not maintain order, but allows access based on a multimap.
- CertificateInfoPair for the elements in the list
◆ subjectPublicKey()
PublicKey QCA::CertificateRequest::subjectPublicKey | ( | ) | const |
The public key belonging to the issuer.
◆ toDER()
QByteArray QCA::CertificateRequest::toDER | ( | ) | const |
Export the Certificate Request into a DER format.
- Note
- this only applies to PKCS#10 format certificate requests
◆ toPEM()
QString QCA::CertificateRequest::toPEM | ( | ) | const |
Export the Certificate Request into a PEM format.
- Note
- this only applies to PKCS#10 format certificate requests
◆ toPEMFile()
bool QCA::CertificateRequest::toPEMFile | ( | const QString & | fileName | ) | const |
Export the Certificate into PEM format in a file.
- Parameters
-
fileName the name of the file to use
- Note
- this only applies to PKCS#10 format certificate requests
◆ toString()
QString QCA::CertificateRequest::toString | ( | ) | const |
Export the CertificateRequest to a string.
- Returns
- the string corresponding to the certificate request
- Note
- this only applies to SPKAC format certificate requests
The documentation for this class was generated from the following file:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Apr 18 2025 12:07:58 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.