Okular::Document
#include <document.h>
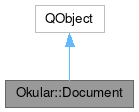
Public Types | |
enum | DocumentAdditionalActionType { CloseDocument , SaveDocumentStart , SaveDocumentFinish , PrintDocumentStart , PrintDocumentFinish } |
enum | MouseEventType { FieldMouseDown , FieldMouseEnter , FieldMouseExit , FieldMouseUp } |
enum | OpenResult { OpenSuccess , OpenError , OpenNeedsPassword } |
enum | PixmapRequestFlag { NoOption = 0 , RemoveAllPrevious = 1 } |
typedef QFlags< PixmapRequestFlag > | PixmapRequestFlags |
enum | PrintError { NoPrintError , UnknownPrintError , TemporaryFileOpenPrintError , FileConversionPrintError , PrintingProcessCrashPrintError , PrintingProcessStartPrintError , PrintToFilePrintError , InvalidPrinterStatePrintError , UnableToFindFilePrintError , NoFileToPrintError , NoBinaryToPrintError , InvalidPageSizePrintError } |
enum | PrintingType { NoPrinting , NativePrinting , PostscriptPrinting } |
enum | SaveCapability { SaveFormsCapability = 1 , SaveAnnotationsCapability = 2 } |
enum | SearchStatus { MatchFound , NoMatchFound , SearchCancelled } |
enum | SearchType { NextMatch , PreviousMatch , AllDocument , GoogleAll , GoogleAny } |
Signals | |
void | aboutToClose () |
void | annotationContentsChangedByUndoRedo (Okular::Annotation *annotation, const QString &contents, int cursorPos, int anchorPos) |
void | canRedoChanged (bool redoAvailable) |
void | canUndoChanged (bool undoAvailable) |
void | close () |
void | error (const QString &text, int duration) |
void | fontReadingEnded () |
void | fontReadingProgress (int page) |
void | formButtonsChangedByUndoRedo (int page, const QList< Okular::FormFieldButton * > &formButtons) |
void | formComboChangedByUndoRedo (int page, Okular::FormFieldChoice *form, const QString &text, int cursorPos, int anchorPos) |
void | formListChangedByUndoRedo (int page, Okular::FormFieldChoice *form, const QList< int > &choices) |
void | formTextChangedByUndoRedo (int page, Okular::FormFieldText *form, const QString &contents, int cursorPos, int anchorPos) |
void | gotFont (const Okular::FontInfo &font) |
void | linkEndPresentation () |
void | linkFind () |
void | linkGoToPage () |
void | linkPresentation () |
void | notice (const QString &text, int duration) |
void | openUrl (const QUrl &url) |
void | processMovieAction (const Okular::MovieAction *action) |
void | processRenditionAction (const Okular::RenditionAction *action) |
void | quit () |
void | refreshFormWidget (Okular::FormField *field) |
void | requestPrint () |
void | requestSaveAs () |
void | searchFinished (int searchID, Okular::Document::SearchStatus endStatus) |
void | sourceReferenceActivated (const QString &absFileName, int line, int col, bool *handled) |
void | undoHistoryCleanChanged (bool clean) |
void | warning (const QString &text, int duration) |
Public Slots | |
void | cancelSearch () |
void | editFormButtons (int pageNumber, const QList< Okular::FormFieldButton * > &formButtons, const QList< bool > &newButtonStates) |
void | editFormCombo (int pageNumber, Okular::FormFieldChoice *form, const QString &newText, int newCursorPos, int prevCursorPos, int prevAnchorPos) |
void | editFormList (int pageNumber, Okular::FormFieldChoice *form, const QList< int > &newChoices) |
OKULARCORE_DEPRECATED void | editFormText (int pageNumber, Okular::FormFieldText *form, const QString &newContents, int newCursorPos, int prevCursorPos, int prevAnchorPos) |
void | editFormText (int pageNumber, Okular::FormFieldText *form, const QString &newContents, int newCursorPos, int prevCursorPos, int prevAnchorPos, const QString &oldContents) |
void | redo () |
void | refreshPixmaps (int pageNumber) |
void | reloadDocument () const |
QByteArray | requestSignedRevisionData (const Okular::SignatureInfo &info) |
void | setPageSize (const Okular::PageSize &size) |
void | setRotation (int rotation) |
void | undo () |
Public Member Functions | |
Document (QWidget *widget) | |
~Document () override | |
void | addObserver (DocumentObserver *observer) |
void | addPageAnnotation (int page, Annotation *annotation) |
void | adjustPageAnnotation (int page, Annotation *annotation, const Okular::NormalizedPoint &delta1, const Okular::NormalizedPoint &delta2) |
QSizeF | allPagesSize () const |
QList< int > | bookmarkedPageList () const |
QString | bookmarkedPageRange () const |
BookmarkManager * | bookmarkManager () const |
bool | canConfigurePrinter () const |
bool | canExportToText () const |
bool | canModifyPageAnnotation (const Annotation *annotation) const |
bool | canProvideFontInformation () const |
bool | canRedo () const |
bool | canRemovePageAnnotation (const Annotation *annotation) const |
bool | canSaveChanges () const |
bool | canSaveChanges (SaveCapability cap) const |
bool | canSign () const |
bool | canSwapBackingFile () const |
bool | canUndo () const |
CertificateStore * | certificateStore () const |
void | clearHistory () |
void | closeDocument () |
int | configurableGenerators () const |
void | continueSearch (int searchID) |
void | continueSearch (int searchID, SearchType type) |
QUrl | currentDocument () const |
uint | currentPage () const |
void | docdataMigrationDone () |
DocumentInfo | documentInfo () const |
DocumentInfo | documentInfo (const QSet< DocumentInfo::Key > &keys) const |
const DocumentSynopsis * | documentSynopsis () const |
const SourceReference * | dynamicSourceReference (int pageNr, double absX, double absY) |
QString | editorCommandOverride () const |
void | editPageAnnotationContents (int page, Annotation *annotation, const QString &newContents, int newCursorPos, int prevCursorPos, int prevAnchorPos) |
const QList< EmbeddedFile * > * | embeddedFiles () const |
QList< ExportFormat > | exportFormats () const |
bool | exportTo (const QString &fileName, const ExportFormat &format) const |
bool | exportToText (const QString &fileName) const |
bool | extractArchivedFile (const QString &destFileName) |
void | fillConfigDialog (KConfigDialog *dialog) |
QByteArray | fontData (const FontInfo &font) const |
KPluginMetaData | generatorInfo () const |
KXMLGUIClient * | guiClient () |
bool | historyAtBegin () const |
bool | historyAtEnd () const |
bool | isAllowed (Permission action) const |
bool | isDocdataMigrationNeeded () const |
bool | isHistoryClean () const |
bool | isOpened () const |
QAbstractItemModel * | layersModel () const |
QVariant | metaData (const QString &key, const QVariant &option=QVariant()) const |
void | modifyPageAnnotationProperties (int page, Annotation *annotation) |
OpenResult | openDocument (const QString &docFile, const QUrl &url, const QMimeType &mime, const QString &password=QString()) |
OpenResult | openDocumentArchive (const QString &docFile, const QUrl &url, const QString &password=QString()) |
QString | openError () const |
QPageLayout::Orientation | orientation () const |
const Page * | page (int number) const |
uint | pages () const |
PageSize::List | pageSizes () const |
QString | pageSizeString (int page) const |
void | prepareToModifyAnnotationProperties (Annotation *annotation) |
Document::PrintError | print (QPrinter &printer) |
QWidget * | printConfigurationWidget () const |
PrintingType | printingSupport () const |
void | processAction (const Action *action) |
void | processDocumentAction (const Action *action, DocumentAdditionalActionType type) |
void | processFocusAction (const Action *action, Okular::FormField *field) |
void | processFormatAction (const Action *action, Okular::FormField *ff) |
OKULARCORE_DEPRECATED void | processFormatAction (const Action *action, Okular::FormFieldText *fft) |
void | processFormMouseScriptAction (const Action *action, Okular::FormField *ff, MouseEventType fieldMouseEventType) |
OKULARCORE_DEPRECATED void | processFormMouseUpScripAction (const Action *action, Okular::FormField *ff) |
void | processKeystrokeAction (const Action *action, Okular::FormField *ff, const QVariant &newValue, int prevCursorPos, int prevAnchorPos) |
OKULARCORE_DEPRECATED void | processKeystrokeAction (const Action *action, Okular::FormFieldText *fft, const QVariant &newValue) |
void | processKeystrokeCommitAction (const Action *action, Okular::FormField *ff, bool &returnCode) |
OKULARCORE_DEPRECATED void | processKeystrokeCommitAction (const Action *action, Okular::FormFieldText *fft) |
void | processKVCFActions (Okular::FormField *ff) |
void | processSourceReference (const SourceReference *reference) |
void | processValidateAction (const Action *action, Okular::FormField *ff, bool &returnCode) |
OKULARCORE_DEPRECATED void | processValidateAction (const Action *action, Okular::FormFieldText *fft, bool &returnCode) |
void | recalculateForms () |
void | registerView (View *view) |
void | removeObserver (DocumentObserver *observer) |
void | removePageAnnotation (int page, Annotation *annotation) |
void | removePageAnnotations (int page, const QList< Annotation * > &annotations) |
void | reparseConfig () |
void | requestPixmaps (const QList< PixmapRequest * > &requests) |
void | requestPixmaps (const QList< PixmapRequest * > &requests, PixmapRequestFlags reqOptions) |
void | requestTextPage (uint pageNumber) |
void | resetSearch (int searchID) |
Rotation | rotation () const |
bool | saveChanges (const QString &fileName) |
bool | saveChanges (const QString &fileName, QString *errorText) |
bool | saveDocumentArchive (const QString &fileName) |
void | searchText (int searchID, const QString &text, bool fromStart, Qt::CaseSensitivity caseSensitivity, SearchType type, bool moveViewport, const QColor &color) |
void | setAnnotationEditingEnabled (bool enable) |
void | setEditorCommandOverride (const QString &editCmd) |
void | setHistoryClean (bool clean) |
void | setNextDocumentDestination (const QString &namedDestination) |
void | setNextDocumentViewport (const DocumentViewport &viewport) |
void | setNextViewport () |
void | setPageTextSelection (int page, std::unique_ptr< RegularAreaRect > &&rect, const QColor &color) |
void | setPrevViewport () |
void | setViewport (const DocumentViewport &viewport, DocumentObserver *excludeObserver=nullptr, bool smoothMove=false, bool updateHistory=true) |
void | setViewportPage (int page, DocumentObserver *excludeObserver=nullptr, bool smoothMove=false) |
void | setVisiblePageRects (const QVector< VisiblePageRect * > &visiblePageRects, DocumentObserver *excludeObserver=nullptr) |
void | setZoom (int factor, DocumentObserver *excludeObserver=nullptr) |
bool | sign (const NewSignatureData &data, const QString &newPath) |
void | startFontReading () |
void | stopFontReading () |
QStringList | supportedMimeTypes () const |
bool | supportsPageSizes () const |
bool | supportsPrintToFile () const |
bool | supportsSearching () const |
bool | supportsTiles () const |
bool | swapBackingFile (const QString &newFileName, const QUrl &url) |
bool | swapBackingFileArchive (const QString &newFileName, const QUrl &url) |
void | translatePageAnnotation (int page, Annotation *annotation, const Okular::NormalizedPoint &delta) |
void | unregisterView (View *view) |
const DocumentViewport & | viewport () const |
const QVector< VisiblePageRect * > & | visiblePageRects () const |
void | walletDataForFile (const QString &fileName, QString *walletName, QString *walletFolder, QString *walletKey) const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Static Public Member Functions | |
static QString | printErrorString (PrintError error) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
The Document.
Heart of everything. Actions take place here.
The Document is the main object in Okular. All views query the Document to get data/properties or even for accessing pages (in a 'const' way).
It is designed to keep it detached from the document type (pdf, ps, you name it..) so whenever you want to get some data, it asks its internal generators to do the job and return results in a format-independent way.
Apart from the generator (the currently running one) the document stores all the Pages ('Page' class) of the current document in a vector and notifies all the registered DocumentObservers when some content changes.
For a better understanding of hierarchies
- See also
- README.internals.png
- DocumentObserver, Page
Definition at line 191 of file document.h.
Member Typedef Documentation
◆ PixmapRequestFlags
Definition at line 477 of file document.h.
Member Enumeration Documentation
◆ DocumentAdditionalActionType
Describes the additional actions available in the Document.
- Since
- 24.08
Definition at line 775 of file document.h.
◆ MouseEventType
Definition at line 756 of file document.h.
◆ OpenResult
Describes the result of an open document operation.
- Since
- 0.20 (KDE 4.14)
Definition at line 210 of file document.h.
◆ PixmapRequestFlag
Describes the possible options for the pixmap requests.
Enumerator | |
---|---|
NoOption | No options. |
RemoveAllPrevious | Remove all the previous requests, even for non requested page pixmaps. |
Definition at line 473 of file document.h.
◆ PrintError
◆ PrintingType
What type of printing a document supports.
Enumerator | |
---|---|
NoPrinting | Printing Not Supported. |
NativePrinting | Native Cross-Platform Printing. |
PostscriptPrinting | Postscript file printing. |
Definition at line 820 of file document.h.
◆ SaveCapability
Saving capabilities.
Their availability varies according to the underlying generator and/or the document type.
- See also
- canSaveChanges (SaveCapability)
- Since
- 0.15 (KDE 4.9)
Enumerator | |
---|---|
SaveFormsCapability | Can save form changes. |
SaveAnnotationsCapability | Can save annotation changes. |
Definition at line 948 of file document.h.
◆ SearchStatus
Describes how search ended.
Enumerator | |
---|---|
MatchFound | Any match was found. |
NoMatchFound | No match was found. |
SearchCancelled | The search was cancelled. |
Definition at line 623 of file document.h.
◆ SearchType
Describes the possible search types.
Definition at line 612 of file document.h.
Constructor & Destructor Documentation
◆ Document()
|
explicit |
Creates a new document with the given widget
as widget to relay GUI things (messageboxes, ...).
Definition at line 2269 of file document.cpp.
◆ ~Document()
|
override |
Destroys the document.
Definition at line 2286 of file document.cpp.
Member Function Documentation
◆ aboutToClose
|
signal |
This signal is emitted whenever the document is about to close.
- Since
- 1.5.3
◆ addObserver()
void Document::addObserver | ( | DocumentObserver * | observer | ) |
Registers a new observer
for the document.
Definition at line 2808 of file document.cpp.
◆ addPageAnnotation()
void Document::addPageAnnotation | ( | int | page, |
Annotation * | annotation ) |
Adds a new annotation
to the given page
.
Definition at line 3515 of file document.cpp.
◆ adjustPageAnnotation()
void Document::adjustPageAnnotation | ( | int | page, |
Annotation * | annotation, | ||
const Okular::NormalizedPoint & | delta1, | ||
const Okular::NormalizedPoint & | delta2 ) |
Adjusts the position of the top-left and bottom-right corners of given annotation
on the given page
.
Can be used to implement resize functionality. delta1
in normalized coordinates is added to top-left. delta2
in normalized coordinates is added to bottom-right.
Consecutive adjustments applied to the same annotation
are merged together on the undo stack if the BeingResized flag is set on the annotation
.
- Since
- 1.1.0
Definition at line 3587 of file document.cpp.
◆ allPagesSize()
QSizeF Document::allPagesSize | ( | ) | const |
If all pages have the same size this method returns it, if the page sizes differ an empty size object is returned.
Definition at line 3238 of file document.cpp.
◆ annotationContentsChangedByUndoRedo
|
signal |
This signal is emitted whenever the contents of the given annotation
are changed by an undo or redo action.
The new contents (contents
), cursor position (cursorPos
), and anchor position (anchorPos
) are included
- Since
- 0.17 (KDE 4.11)
◆ bookmarkedPageList()
QList< int > Document::bookmarkedPageList | ( | ) | const |
Returns a list of the bookmarked.pages.
Definition at line 4065 of file document.cpp.
◆ bookmarkedPageRange()
QString Document::bookmarkedPageRange | ( | ) | const |
Returns the range of the bookmarked.pages.
Definition at line 4079 of file document.cpp.
◆ bookmarkManager()
BookmarkManager * Document::bookmarkManager | ( | ) | const |
Returns the bookmark manager of the document.
Definition at line 4060 of file document.cpp.
◆ cancelSearch
|
slot |
Cancels the current search.
Definition at line 3996 of file document.cpp.
◆ canConfigurePrinter()
bool Document::canConfigurePrinter | ( | ) | const |
Returns whether the document can configure the printer itself.
Definition at line 2891 of file document.cpp.
◆ canExportToText()
bool Document::canExportToText | ( | ) | const |
Returns whether the document supports the export to ASCII text.
Definition at line 3119 of file document.cpp.
◆ canModifyPageAnnotation()
bool Document::canModifyPageAnnotation | ( | const Annotation * | annotation | ) | const |
Tests if the annotation
can be modified.
- Since
- 0.15 (KDE 4.9)
Definition at line 3525 of file document.cpp.
◆ canProvideFontInformation()
bool Document::canProvideFontInformation | ( | ) | const |
Whether the current document can provide information about the fonts used in it.
Definition at line 3014 of file document.cpp.
◆ canRedo()
bool Document::canRedo | ( | ) | const |
Returns true if there is a redo command available; otherwise returns false.
- Since
- 0.17 (KDE 4.11)
Definition at line 3697 of file document.cpp.
◆ canRedoChanged
|
signal |
This signal is emitted whenever the availability of the redo function changes.
- Since
- 0.17 (KDE 4.11)
◆ canRemovePageAnnotation()
bool Document::canRemovePageAnnotation | ( | const Annotation * | annotation | ) | const |
Tests if the annotation
can be removed.
- Since
- 0.15 (KDE 4.9)
Definition at line 3601 of file document.cpp.
◆ canSaveChanges() [1/2]
bool Document::canSaveChanges | ( | ) | const |
Returns whether the changes to the document (modified annotations, values in form fields, etc) can be saved to another document.
Equivalent to the logical OR of canSaveChanges(SaveCapability) for each capability.
- Since
- 0.7 (KDE 4.1)
Definition at line 5171 of file document.cpp.
◆ canSaveChanges() [2/2]
bool Document::canSaveChanges | ( | SaveCapability | cap | ) | const |
Returns whether it's possible to save a given category of changes to another document.
- Since
- 0.15 (KDE 4.9)
Definition at line 5188 of file document.cpp.
◆ canSign()
bool Document::canSign | ( | ) | const |
Whether the current document can perform digital signing.
Definition at line 3019 of file document.cpp.
◆ canSwapBackingFile()
bool Document::canSwapBackingFile | ( | ) | const |
Returns whether the generator supports hot-swapping the current file with another identical file.
- Since
- 1.3
Definition at line 5024 of file document.cpp.
◆ canUndo()
bool Document::canUndo | ( | ) | const |
Returns true if there is an undo command available; otherwise returns false.
- Since
- 0.17 (KDE 4.11)
Definition at line 3692 of file document.cpp.
◆ canUndoChanged
|
signal |
This signal is emitted whenever the availability of the undo function changes.
- Since
- 0.17 (KDE 4.11)
◆ certificateStore()
Okular::CertificateStore * Document::certificateStore | ( | ) | const |
Returns the generator's certificate store (if any)
- Since
- 21.04
Definition at line 2910 of file document.cpp.
◆ clearHistory()
void Document::clearHistory | ( | ) |
- Since
- 24.12
Definition at line 5166 of file document.cpp.
◆ close
|
signal |
This signal is emitted whenever an action requests a document close operation.
◆ closeDocument()
void Document::closeDocument | ( | ) |
Closes the document.
Definition at line 2641 of file document.cpp.
◆ configurableGenerators()
int Document::configurableGenerators | ( | ) | const |
Returns the number of generators that have a configuration widget.
Definition at line 4986 of file document.cpp.
◆ continueSearch() [1/2]
void Document::continueSearch | ( | int | searchID | ) |
Continues the search for the given searchID
.
Definition at line 3934 of file document.cpp.
◆ continueSearch() [2/2]
void Document::continueSearch | ( | int | searchID, |
SearchType | type ) |
Continues the search for the given searchID
, optionally specifying a new type for the search.
- Since
- 0.7 (KDE 4.1)
Definition at line 3950 of file document.cpp.
◆ currentDocument()
QUrl Document::currentDocument | ( | ) | const |
Returns the url of the currently opened document.
Definition at line 3070 of file document.cpp.
◆ currentPage()
uint Document::currentPage | ( | ) | const |
Returns the number of the current page.
Definition at line 3060 of file document.cpp.
◆ docdataMigrationDone()
void Document::docdataMigrationDone | ( | ) |
Delete annotations and form data from the docdata folder.
Call it if isDocdataMigrationNeeded() was true and you've just saved them to an external file.
- Since
- 1.3
Definition at line 5552 of file document.cpp.
◆ documentInfo() [1/2]
DocumentInfo Okular::Document::documentInfo | ( | ) | const |
Returns the meta data of the document.
◆ documentInfo() [2/2]
DocumentInfo Document::documentInfo | ( | const QSet< DocumentInfo::Key > & | keys | ) | const |
Returns the asked set of meta data of the document.
The result may contain more metadata than the one asked for.
Definition at line 2935 of file document.cpp.
◆ documentSynopsis()
const DocumentSynopsis * Document::documentSynopsis | ( | ) | const |
Returns the table of content of the document or 0 if no table of content is available.
Definition at line 2972 of file document.cpp.
◆ dynamicSourceReference()
const SourceReference * Document::dynamicSourceReference | ( | int | pageNr, |
double | absX, | ||
double | absY ) |
Asks the generator to dynamically generate a SourceReference for a given page number and absolute X and Y position on this page.
- Attention
- Ownership of the returned SourceReference is transferred to the caller.
- Note
- This method does not call processSourceReference( const SourceReference * )
- Since
- 0.10 (KDE 4.4)
Definition at line 4812 of file document.cpp.
◆ editFormButtons
|
slot |
Set the states of the group of form buttons formButtons
on page page
to newButtonStates
.
The lists formButtons
and newButtonStates
should be the same length and true values in newButtonStates
indicate that the corresponding entry in formButtons
should be enabled.
Definition at line 4043 of file document.cpp.
◆ editFormCombo
|
slot |
Set the active choice in the combo box form
on page page
to newText
The new cursor position (newCursorPos
), previous cursor position (prevCursorPos
), and previous anchor position (prevAnchorPos
) will be restored by the undo / redo commands.
- Since
- 0.17 (KDE 4.11)
Definition at line 4030 of file document.cpp.
◆ editFormList
|
slot |
Edit the selected list entries in form
on page page
to be newChoices
.
- Since
- 0.17 (KDE 4.11)
Definition at line 4023 of file document.cpp.
◆ editFormText [1/2]
|
slot |
Edit the text contents of the specified form
on page page
to be newContents
.
The new text cursor position (newCursorPos
), previous text cursor position (prevCursorPos
), and previous cursor anchor position will be restored by the undo / redo commands.
- Since
- 0.17 (KDE 4.11)
- Deprecated
- use editFormText(int pageNumber, Okular::FormFieldText *form, const QString &newContents, int newCursorPos, int prevCursorPos, int prevAnchorPos, const QString &oldContents)
Definition at line 4011 of file document.cpp.
◆ editFormText [2/2]
|
slot |
Edit the text contents of the specified form
on page page
to be newContents
where previous contents are oldContents
for undo/redo commands.
The new text cursor position (newCursorPos
), previous text cursor position (prevCursorPos
), and previous cursor anchor position will be restored by the undo / redo commands.
- Since
- 24.08
Definition at line 4017 of file document.cpp.
◆ editorCommandOverride()
QString Document::editorCommandOverride | ( | ) | const |
returns the overriding editor command.
If the editor command was not overriden, the string is empty.
- Since
- 22.04
Definition at line 2920 of file document.cpp.
◆ editPageAnnotationContents()
void Document::editPageAnnotationContents | ( | int | page, |
Annotation * | annotation, | ||
const QString & | newContents, | ||
int | newCursorPos, | ||
int | prevCursorPos, | ||
int | prevAnchorPos ) |
Edits the plain text contents of the given annotation
on the given page
.
The contents are set to newContents
with cursor position newCursorPos
. The previous cursor position prevCursorPos
and previous anchor position prevAnchorPos
must also be supplied so that they can be restored if the edit action is undone.
The Annotation's internal contents should not be modified prior to calling this method.
- Since
- 0.17 (KDE 4.11)
Definition at line 3594 of file document.cpp.
◆ embeddedFiles()
const QList< EmbeddedFile * > * Document::embeddedFiles | ( | ) | const |
Returns the list of embedded files or 0 if no embedded files are available.
Definition at line 3024 of file document.cpp.
◆ error
|
signal |
This signal is emitted whenever an error occurred.
- Parameters
-
text The description of the error. duration The time in milliseconds the message should be shown to the user.
◆ exportFormats()
ExportFormat::List Document::exportFormats | ( | ) | const |
Returns the list of supported export formats.
- See also
- ExportFormat
Definition at line 3143 of file document.cpp.
◆ exportTo()
bool Document::exportTo | ( | const QString & | fileName, |
const ExportFormat & | format ) const |
Exports the document in the given format
and saves it under fileName
.
Definition at line 3153 of file document.cpp.
◆ exportToText()
bool Document::exportToText | ( | const QString & | fileName | ) | const |
Exports the document as ASCII text and saves it under fileName
.
Definition at line 3129 of file document.cpp.
◆ extractArchivedFile()
bool Document::extractArchivedFile | ( | const QString & | destFileName | ) |
Extract the document file from the current archive.
- Warning
- This function only works if the current file is a document archive
- Since
- 1.3
Definition at line 5496 of file document.cpp.
◆ fillConfigDialog()
void Document::fillConfigDialog | ( | KConfigDialog * | dialog | ) |
Fill the KConfigDialog dialog
with the setting pages of the generators.
Definition at line 4916 of file document.cpp.
◆ fontData()
QByteArray Document::fontData | ( | const FontInfo & | font | ) | const |
Gets the font data for the given font.
- Since
- 0.8 (KDE 4.2)
Definition at line 5272 of file document.cpp.
◆ fontReadingEnded
|
signal |
Reports that the reading of the fonts in the document is finished.
◆ fontReadingProgress
|
signal |
Reports the progress when reading the fonts in the document.
- Parameters
-
page is the page that was just finished to scan for fonts
◆ formButtonsChangedByUndoRedo
|
signal |
This signal is emitted whenever the state of the specified group of form buttons (formButtons
) on the given page
is changed by an undo or redo action.
- Since
- 0.17 (KDE 4.11)
◆ formComboChangedByUndoRedo
|
signal |
This signal is emitted whenever the active text
for the given combo form
on the given page
is changed by an undo or redo action.
- Since
- 0.17 (KDE 4.11)
◆ formListChangedByUndoRedo
|
signal |
This signal is emitted whenever the selected choices
for the given list form
on the given page
are changed by an undo or redo action.
- Since
- 0.17 (KDE 4.11)
◆ formTextChangedByUndoRedo
|
signal |
This signal is emitted whenever the text contents of the given text form
on the given page
are changed by an undo or redo action.
The new text contents (contents
), cursor position (cursorPos
), and anchor position (anchorPos
) are included
- Since
- 0.17 (KDE 4.11)
◆ generatorInfo()
KPluginMetaData Document::generatorInfo | ( | ) | const |
Returns the metadata associated with the generator.
May be invalid.
Definition at line 4975 of file document.cpp.
◆ gotFont
|
signal |
Emitted when a new font is found during the reading of the fonts of the document.
◆ guiClient()
KXMLGUIClient * Document::guiClient | ( | ) |
Returns the gui client of the generator, if it provides one.
Definition at line 2630 of file document.cpp.
◆ historyAtBegin()
bool Document::historyAtBegin | ( | ) | const |
Returns whether the document history is at the begin.
Definition at line 3158 of file document.cpp.
◆ historyAtEnd()
bool Document::historyAtEnd | ( | ) | const |
Returns whether the document history is at the end.
Definition at line 3163 of file document.cpp.
◆ isAllowed()
bool Document::isAllowed | ( | Permission | action | ) | const |
Returns whether the given action
is allowed in the document.
- See also
- Permission
Definition at line 3075 of file document.cpp.
◆ isDocdataMigrationNeeded()
bool Document::isDocdataMigrationNeeded | ( | ) | const |
Since version 0.21, okular does not allow editing annotations and form data if they are stored in the docdata directory (like older okular versions did by default).
If this flag is set, then annotations and forms cannot be edited.
- Since
- 1.3
Definition at line 5547 of file document.cpp.
◆ isHistoryClean()
bool Document::isHistoryClean | ( | ) | const |
Definition at line 5161 of file document.cpp.
◆ isOpened()
bool Document::isOpened | ( | ) | const |
Returns whether the document is currently opened.
Definition at line 2886 of file document.cpp.
◆ layersModel()
QAbstractItemModel * Document::layersModel | ( | ) | const |
Returns the model for rendering layers (NULL if the document has no layers)
- Since
- 0.24
Definition at line 5560 of file document.cpp.
◆ linkEndPresentation
|
signal |
This signal is emitted whenever an action requests an end presentation operation.
◆ linkFind
|
signal |
This signal is emitted whenever an action requests a find operation.
◆ linkGoToPage
|
signal |
This signal is emitted whenever an action requests a goto operation.
◆ linkPresentation
|
signal |
This signal is emitted whenever an action requests a start presentation operation.
◆ metaData()
Returns the meta data for the given key
and option
or an empty variant if the key doesn't exists.
Definition at line 3168 of file document.cpp.
◆ modifyPageAnnotationProperties()
void Document::modifyPageAnnotationProperties | ( | int | page, |
Annotation * | annotation ) |
Modifies the given annotation
on the given page
.
Must be preceded by a call to prepareToModifyAnnotationProperties before the annotation's properties are modified
- Since
- 0.17 (KDE 4.11)
Definition at line 3567 of file document.cpp.
◆ notice
|
signal |
This signal is emitted to signal a notice.
- Parameters
-
text The description of the notice. duration The time in milliseconds the message should be shown to the user.
◆ openDocument()
◆ openDocumentArchive()
Document::OpenResult Document::openDocumentArchive | ( | const QString & | docFile, |
const QUrl & | url, | ||
const QString & | password = QString() ) |
◆ openError()
QString Document::openError | ( | ) | const |
Returns the reason why the file opening failed, if any.
- Since
- 1.10
Definition at line 5565 of file document.cpp.
◆ openUrl
|
signal |
This signal is emitted whenever an action requests an open url operation for the given document url
.
◆ orientation()
QPageLayout::Orientation Document::orientation | ( | ) | const |
Returns the orientation of the document (for printing purposes).
This is used in the KPart to initialize the print dialog and in the generators to check whether the document needs to be rotated or not.
- Since
- 0.14 (KDE 4.8)
Definition at line 5508 of file document.cpp.
◆ page()
const Page * Document::page | ( | int | number | ) | const |
Returns the page object for the given page number
or 0 if the number is out of range.
Definition at line 3029 of file document.cpp.
◆ pages()
uint Document::pages | ( | ) | const |
Returns the number of pages of the document.
Definition at line 3065 of file document.cpp.
◆ pageSizes()
PageSize::List Document::pageSizes | ( | ) | const |
Returns the list of supported page sizes or an empty list if this feature is not available.
- See also
- supportsPageSizes()
Definition at line 3108 of file document.cpp.
◆ pageSizeString()
QString Document::pageSizeString | ( | int | page | ) | const |
Returns the size string for the given page
or an empty string if the page is out of range.
Definition at line 3257 of file document.cpp.
◆ prepareToModifyAnnotationProperties()
void Document::prepareToModifyAnnotationProperties | ( | Annotation * | annotation | ) |
Prepares to modify the properties of the given annotation
.
Must be called before the annotation's properties are modified
- Since
- 0.17 (KDE 4.11)
Definition at line 3557 of file document.cpp.
◆ print()
Document::PrintError Document::print | ( | QPrinter & | printer | ) |
Prints the document to the given printer
.
Definition at line 4860 of file document.cpp.
◆ printConfigurationWidget()
QWidget * Document::printConfigurationWidget | ( | ) | const |
Returns a custom printer configuration page or 0 if no custom printer configuration page is available.
The returned object should be of a PrintOptionsWidget subclass (which is not officially enforced by the signature for binary compatibility reasons).
Definition at line 4906 of file document.cpp.
◆ printErrorString()
|
static |
- Since
- 22.04
Definition at line 4874 of file document.cpp.
◆ printingSupport()
Document::PrintingType Document::printingSupport | ( | ) | const |
Returns what sort of printing the document supports: Native, Postscript, None.
Definition at line 4838 of file document.cpp.
◆ processAction()
void Document::processAction | ( | const Action * | action | ) |
Processes the given action
.
Definition at line 4158 of file document.cpp.
◆ processDocumentAction()
void Document::processDocumentAction | ( | const Action * | action, |
DocumentAdditionalActionType | type ) |
Processes the given document additional action
of specified type
.
- Since
- 24.08
Definition at line 4662 of file document.cpp.
◆ processFocusAction()
void Document::processFocusAction | ( | const Action * | action, |
Okular::FormField * | field ) |
Processes the given focus action on the field.
- Since
- 1.9
Definition at line 4571 of file document.cpp.
◆ processFormatAction() [1/2]
void Document::processFormatAction | ( | const Action * | action, |
Okular::FormField * | ff ) |
Processes the given format action
on a Form Field ff
.
- Since
- 24.08
Definition at line 4385 of file document.cpp.
◆ processFormatAction() [2/2]
void Document::processFormatAction | ( | const Action * | action, |
Okular::FormFieldText * | fft ) |
◆ processFormMouseScriptAction()
void Document::processFormMouseScriptAction | ( | const Action * | action, |
Okular::FormField * | ff, | ||
MouseEventType | fieldMouseEventType ) |
Processes the mouse action
of type fieldMouseEventType
on ff
.
- Since
- 24.12
Definition at line 4695 of file document.cpp.
◆ processFormMouseUpScripAction()
void Document::processFormMouseUpScripAction | ( | const Action * | action, |
Okular::FormField * | ff ) |
◆ processKeystrokeAction() [1/2]
void Document::processKeystrokeAction | ( | const Action * | action, |
Okular::FormField * | ff, | ||
const QVariant & | newValue, | ||
int | prevCursorPos, | ||
int | prevAnchorPos ) |
Processes the given keystroke action
on ff
between the two positions prevCursorPos
and prevAnchorPos
prevCursorPos
and prevAnchorPos
are used to set the selStart and selEnd event properties.
- Since
- 24.08
Definition at line 4455 of file document.cpp.
◆ processKeystrokeAction() [2/2]
void Document::processKeystrokeAction | ( | const Action * | action, |
Okular::FormFieldText * | fft, | ||
const QVariant & | newValue ) |
Processes the given keystroke action
on fft
.
- Since
- 1.9
- Deprecated
- use processKeystrokeAction(const Action *, Okular::FormField *, const QVariant &, int, int)
Definition at line 4527 of file document.cpp.
◆ processKeystrokeCommitAction() [1/2]
void Document::processKeystrokeCommitAction | ( | const Action * | action, |
Okular::FormField * | ff, | ||
bool & | returnCode ) |
Processes the given keystroke action
on FormField ff
.
This will set event.willCommit=true. The return code parameter is set if the value is to be committed.
- Since
- 24.08
Definition at line 4539 of file document.cpp.
◆ processKeystrokeCommitAction() [2/2]
void Document::processKeystrokeCommitAction | ( | const Action * | action, |
Okular::FormFieldText * | fft ) |
Processes the given keystroke action
on fft
.
This will set event.willCommit=true
- Since
- 22.04
Definition at line 4533 of file document.cpp.
◆ processKVCFActions()
void Document::processKVCFActions | ( | Okular::FormField * | ff | ) |
A method that executes the relevant keystroke, validate, calculate and format actions on a FormField ff
.
- Since
- 24.08
Definition at line 4627 of file document.cpp.
◆ processMovieAction
|
signal |
This signal is emitted whenever an movie action is triggered and the UI should process it.
◆ processRenditionAction
|
signal |
This signal is emitted whenever an rendition action is triggered and the UI should process it.
- Since
- 0.16 (KDE 4.10)
◆ processSourceReference()
void Document::processSourceReference | ( | const SourceReference * | reference | ) |
Processes/Executes the given source reference
.
Definition at line 4738 of file document.cpp.
◆ processValidateAction() [1/2]
void Document::processValidateAction | ( | const Action * | action, |
Okular::FormField * | ff, | ||
bool & | returnCode ) |
Validates the input value in the FormField ff
and sets the returnCode
for a given validate action
.
- Since
- 24.08
Definition at line 4597 of file document.cpp.
◆ processValidateAction() [2/2]
void Document::processValidateAction | ( | const Action * | action, |
Okular::FormFieldText * | fft, | ||
bool & | returnCode ) |
◆ quit
|
signal |
This signal is emitted whenever an action requests an application quit operation.
◆ recalculateForms()
void Document::recalculateForms | ( | ) |
Recalculates all the form fields in the document.
- Since
- 24.12
Definition at line 3510 of file document.cpp.
◆ redo
|
slot |
◆ refreshFormWidget
|
signal |
This signal is emitted whenever a FormField was changed programmatically and the according widget should be updated.
- Since
- 1.4
◆ refreshPixmaps
|
slot |
Refresh the pixmaps for the given pageNumber
.
- Since
- 1.10
Definition at line 5586 of file document.cpp.
◆ registerView()
void Document::registerView | ( | View * | view | ) |
Register the specified view
for the current document.
It is unregistered from the previous document, if any.
- Since
- 0.7 (KDE 4.1)
Definition at line 5237 of file document.cpp.
◆ reloadDocument
|
slot |
◆ removeObserver()
void Document::removeObserver | ( | DocumentObserver * | observer | ) |
Unregisters the given observer
for the document.
Definition at line 2820 of file document.cpp.
◆ removePageAnnotation()
void Document::removePageAnnotation | ( | int | page, |
Annotation * | annotation ) |
Removes the given annotation
from the given page
.
Definition at line 3625 of file document.cpp.
◆ removePageAnnotations()
void Document::removePageAnnotations | ( | int | page, |
const QList< Annotation * > & | annotations ) |
Removes the given annotations
from the given page
.
Definition at line 3631 of file document.cpp.
◆ reparseConfig()
void Document::reparseConfig | ( | ) |
Reparses and applies the configuration.
Definition at line 2854 of file document.cpp.
◆ requestPixmaps() [1/2]
void Document::requestPixmaps | ( | const QList< PixmapRequest * > & | requests | ) |
Sends requests
for pixmap generation.
The same as requestPixmaps( requests, RemoveAllPrevious );
- Since
- 22.08
Definition at line 3351 of file document.cpp.
◆ requestPixmaps() [2/2]
void Document::requestPixmaps | ( | const QList< PixmapRequest * > & | requests, |
PixmapRequestFlags | reqOptions ) |
Sends requests
for pixmap generation.
- Parameters
-
requests the linked list of requests reqOptions the options for the request
- Since
- 22.08
Definition at line 3356 of file document.cpp.
◆ requestPrint
|
signal |
This signal is emitted whenever an action requests a document print operation.
- Since
- 22.04
◆ requestSaveAs
|
signal |
This signal is emitted whenever an action requests a document save as operation.
- Since
- 22.04
◆ requestSignedRevisionData
|
slot |
Returns the part of document covered by the given signature info
.
- Since
- 1.7
Definition at line 5570 of file document.cpp.
◆ requestTextPage()
void Document::requestTextPage | ( | uint | pageNumber | ) |
Sends a request for text page generation for the given page pageNumber
.
Definition at line 3488 of file document.cpp.
◆ resetSearch()
void Document::resetSearch | ( | int | searchID | ) |
Resets the search for the given searchID
.
Definition at line 3966 of file document.cpp.
◆ rotation()
Rotation Document::rotation | ( | ) | const |
Returns the current rotation of the document.
Definition at line 3233 of file document.cpp.
◆ saveChanges() [1/2]
bool Document::saveChanges | ( | const QString & | fileName | ) |
Save the document and the optional changes to it to the specified fileName
.
- Since
- 0.7 (KDE 4.1)
Definition at line 5204 of file document.cpp.
◆ saveChanges() [2/2]
Save the document and the optional changes to it to the specified fileName
and returns a errorText
if fails.
- Since
- 0.10 (KDE 4.4)
Definition at line 5210 of file document.cpp.
◆ saveDocumentArchive()
bool Document::saveDocumentArchive | ( | const QString & | fileName | ) |
◆ searchFinished
|
signal |
Reports that the current search finished.
◆ searchText()
void Document::searchText | ( | int | searchID, |
const QString & | text, | ||
bool | fromStart, | ||
Qt::CaseSensitivity | caseSensitivity, | ||
SearchType | type, | ||
bool | moveViewport, | ||
const QColor & | color ) |
Searches the given text
in the document.
- Parameters
-
searchID The unique id for this search request. text The text to be searched. fromStart Whether the search should be started at begin of the document. caseSensitivity Whether the search is case sensitive. type The type of the search. SearchType moveViewport Whether the viewport shall be moved to the position of the matches. color The highlighting color of the matches.
Definition at line 3837 of file document.cpp.
◆ setAnnotationEditingEnabled()
void Document::setAnnotationEditingEnabled | ( | bool | enable | ) |
Control annotation editing (creation, modification and removal), which is enabled by default.
- Since
- 0.15 (KDE 4.9)
Definition at line 5532 of file document.cpp.
◆ setEditorCommandOverride()
void Document::setEditorCommandOverride | ( | const QString & | editCmd | ) |
sets the editor command to the command editCmd
, as given at the commandline.
- Since
- 22.04
Definition at line 2915 of file document.cpp.
◆ setHistoryClean()
void Document::setHistoryClean | ( | bool | clean | ) |
◆ setNextDocumentDestination()
void Document::setNextDocumentDestination | ( | const QString & | namedDestination | ) |
Sets the next namedDestination
in the viewport history.
- Since
- 0.9 (KDE 4.3)
Definition at line 3832 of file document.cpp.
◆ setNextDocumentViewport()
void Document::setNextDocumentViewport | ( | const DocumentViewport & | viewport | ) |
Sets the next viewport
in the viewport history.
Definition at line 3827 of file document.cpp.
◆ setNextViewport()
void Document::setNextViewport | ( | ) |
Sets the current document viewport to the previous viewport in the viewport history.
Definition at line 3809 of file document.cpp.
◆ setPageSize
|
slot |
This slot is called whenever the user changes the page size
of the document.
Definition at line 5770 of file document.cpp.
◆ setPageTextSelection()
void Document::setPageTextSelection | ( | int | page, |
std::unique_ptr< RegularAreaRect > && | rect, | ||
const QColor & | color ) |
Clears the text selection highlights for the given page
, creates new ones if rect
is not nullptr, and deletes rect
.
- Parameters
-
page The number of the page. rect The rectangle of the selection. color The color of the selection.
Definition at line 3674 of file document.cpp.
◆ setPrevViewport()
void Document::setPrevViewport | ( | ) |
Sets the current document viewport to the next viewport in the viewport history.
Definition at line 3793 of file document.cpp.
◆ setRotation
|
slot |
This slot is called whenever the user changes the rotation
of the document.
Definition at line 5738 of file document.cpp.
◆ setViewport()
void Document::setViewport | ( | const DocumentViewport & | viewport, |
DocumentObserver * | excludeObserver = nullptr, | ||
bool | smoothMove = false, | ||
bool | updateHistory = true ) |
Sets the current document viewport to the given viewport
.
- Parameters
-
viewport The document viewport. excludeObserver The observer which shouldn't be effected by this change. smoothMove Whether the move shall be animated smoothly. updateHistory Whether to consider the change of viewport for the history navigation
Definition at line 3718 of file document.cpp.
◆ setViewportPage()
void Document::setViewportPage | ( | int | page, |
DocumentObserver * | excludeObserver = nullptr, | ||
bool | smoothMove = false ) |
Sets the current document viewport to the given page
.
- Parameters
-
page The number of the page. excludeObserver The observer ids which shouldn't be effected by this change. smoothMove Whether the move shall be animated smoothly.
Definition at line 3770 of file document.cpp.
◆ setVisiblePageRects()
void Document::setVisiblePageRects | ( | const QVector< VisiblePageRect * > & | visiblePageRects, |
DocumentObserver * | excludeObserver = nullptr ) |
Sets the list of visible page rectangles.
- See also
- VisiblePageRect
Definition at line 3044 of file document.cpp.
◆ setZoom()
void Document::setZoom | ( | int | factor, |
DocumentObserver * | excludeObserver = nullptr ) |
Sets the zoom for the current document.
Definition at line 3783 of file document.cpp.
◆ sign()
bool Document::sign | ( | const NewSignatureData & | data, |
const QString & | newPath ) |
◆ sourceReferenceActivated
|
signal |
This signal is emitted whenever a source reference with the given parameters has been activated.
- Parameters
-
absFileName absolute name of the file. line line number. col column number. handled should be set to 'true' if a slot handles this source reference; the default action to launch the configured editor will then not be performed by the document
- Since
- 0.14 (KDE 4.8)
◆ startFontReading()
void Document::startFontReading | ( | ) |
Starts the reading of the information about the fonts in the document, if available.
The results as well the end of the reading is notified using the signals gotFont(), fontReadingProgress() and fontReadingEnded()
Definition at line 2977 of file document.cpp.
◆ stopFontReading()
void Document::stopFontReading | ( | ) |
Force the termination of the reading of the information about the fonts in the document, if running.
Definition at line 3002 of file document.cpp.
◆ supportedMimeTypes()
QStringList Document::supportedMimeTypes | ( | ) | const |
Returns the list with the supported MIME types.
Definition at line 4991 of file document.cpp.
◆ supportsPageSizes()
bool Document::supportsPageSizes | ( | ) | const |
Returns whether the document supports the listing of page sizes.
Definition at line 3098 of file document.cpp.
◆ supportsPrintToFile()
bool Document::supportsPrintToFile | ( | ) | const |
Returns whether the document supports printing to both PDF and PS files.
Definition at line 4855 of file document.cpp.
◆ supportsSearching()
bool Document::supportsSearching | ( | ) | const |
Returns whether the document supports searching.
Definition at line 3093 of file document.cpp.
◆ supportsTiles()
bool Document::supportsTiles | ( | ) | const |
Returns whether the current document supports tiles.
- Since
- 0.16 (KDE 4.10)
Definition at line 3103 of file document.cpp.
◆ swapBackingFile()
Reload the document from a new location, without any visible effect to the user.
The new file must be identical to the current one or, if the document has been modified (eg the user edited forms and annotations), the new document must have these changes too. For example, you can call saveChanges first to write changes to a file and then swapBackingFile to switch to the new location.
- Since
- 1.3
Definition at line 5033 of file document.cpp.
◆ swapBackingFileArchive()
Same as swapBackingFile, but newFileName must be a .okular file.
The new file must be identical to the current one or, if the document has been modified (eg the user edited forms and annotations), the new document must have these changes too. For example, you can call saveDocumentArchive first to write changes to a file and then swapBackingFileArchive to switch to the new location.
- Since
- 1.3
Definition at line 5131 of file document.cpp.
◆ translatePageAnnotation()
void Document::translatePageAnnotation | ( | int | page, |
Annotation * | annotation, | ||
const Okular::NormalizedPoint & | delta ) |
Translates the position of the given annotation
on the given page
by a distance delta
in normalized coordinates.
Consecutive translations applied to the same annotation
are merged together on the undo stack if the BeingMoved flag is set on the annotation
.
- Since
- 0.17 (KDE 4.11)
Definition at line 3580 of file document.cpp.
◆ undo
|
slot |
◆ undoHistoryCleanChanged
|
signal |
This signal is emitted whenever the undo history is clean (i.e.
the same status the last time it was saved)
- Since
- 1.3
◆ unregisterView()
void Document::unregisterView | ( | View * | view | ) |
Unregister the specified view
from the current document.
- Since
- 0.7 (KDE 4.1)
Definition at line 5257 of file document.cpp.
◆ viewport()
const DocumentViewport & Document::viewport | ( | ) | const |
Returns the current viewport of the document.
Definition at line 3034 of file document.cpp.
◆ visiblePageRects()
const QVector< VisiblePageRect * > & Document::visiblePageRects | ( | ) | const |
Returns the list of visible page rectangles.
Definition at line 3039 of file document.cpp.
◆ walletDataForFile()
void Document::walletDataForFile | ( | const QString & | fileName, |
QString * | walletName, | ||
QString * | walletFolder, | ||
QString * | walletKey ) const |
Returns which wallet data to use to read/write the password for the given fileName.
- Since
- 0.20 (KDE 4.14)
Definition at line 5538 of file document.cpp.
◆ warning
|
signal |
This signal is emitted to signal a warning.
- Parameters
-
text The description of the warning. duration The time in milliseconds the message should be shown to the user.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 11:59:13 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.